One of the new features in ASP.NET MVC 3 is the [SessionState] Attribute that can be decorated on controllers. Using the attribute, you can completely turn on or off session state, adjust it to readonly, or make it required using a SessionStateBehavior Enum with the following choices:
- Default - The default ASP.NET logic is used to determine the session state behavior for the request. The default logic looks for the existence of marker session state interfaces on the IHttpHandler.
- Required - Full read-write session state behavior is enabled for the request. This setting overrides whatever session behavior would have been determined by inspecting the handler for the request.
- ReadOnly - Read-only session state is enabled for the request. This means that session state cannot be updated. This setting overrides whatever session state behavior would have been determined by inspecting the handler for the request.
- Disabled - Session state is not enabled for processing the request. This setting overrides whatever session behavior would have been determined by inspecting the handler for the request.
Turning off session state using the new SessionState Attribute in ASP.NET MVC 3 would look like this:
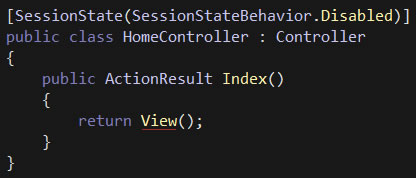
Obviously if session state is disabled we should no longer try to use the Session Property on the Controller as it will be null. Turning off session state and using the Session Property will give you the dreaded “object reference not set to an instance of object” error:
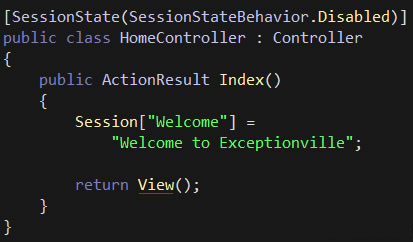
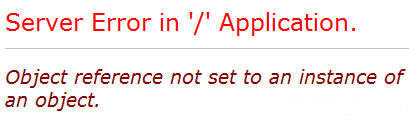
TempData and Session State
One thing that might not be so obvious is that the default provider for TempData uses session state ( SessionStateTempDataProvider ). Therefore if you turn off session state for the request but then try to access TempData you will get an error. Here is the use of TempData and the default SessionStateTempDataProvider:
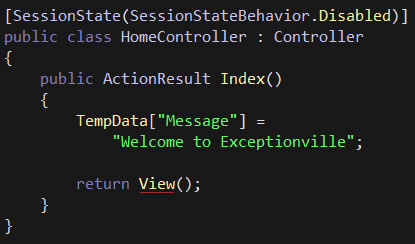
and here is “The SessionStateTempDataProvider class requires session state to be enabled” exception you will receive:
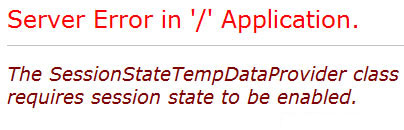
If you still want to disable session state but use TempData, create a different provider for it that uses browser cookies for example. As it so happens, you can find a CookieTempDataProvider in ASP.NET MVC 3 Futures
Session State and Child Actions
The TempData scenario may be obvious, but this child action scenario may not be so obvious. Let's say we turned off session state in the parent controller and then we call a child action in a different controller using Html.RenderAction or Html.Action from within the view.

Does the child action have access to TempData and Session? The Child Controller looks like this:
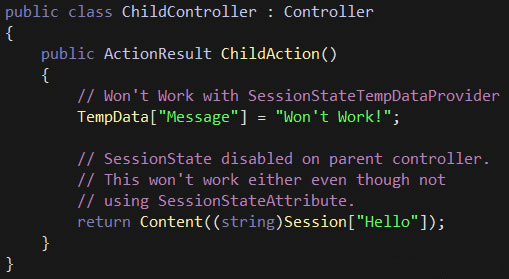
Even though we did not disable session state on this controller, we won't have access to Session State or TempData in the child action because session state was disabled in the parent controller. The same errors mentioned above will occur.
Child Actions vs. Ajax Requests
Don't confuse ajax requests with child actions. I don't suspect you would, but the question has come up before. A child action is kicked off using Html.RenderAction or Html.Action in a view. Ajax requests coming from the browser are not child actions, but completely separate requests into the application. If you use jQuery within a view to grab data from the same action used above, called ChildAction, the action will have full access to session state since the request is no longer a child action but a normal request coming into the application.
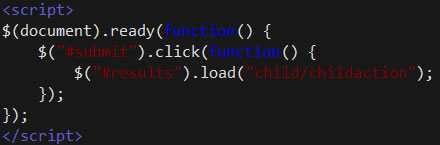
I changed the controller to just grab the SessionId which will work fine:
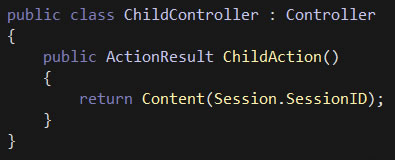
Of course, this is true of any direct request to the action (e.g. http://.../child/childaction ). That request would no longer be a child action, but a regular ol' request. Since it is a regular request through this controller and session state has not been disabled using the [SessionState] Attribute or other means, session state is enabled and fully accessible.
Why Bother With SessionLess Controllers
The big question becomes why bother with SessionLess Controllers in ASP.NET MVC 3 if we have to tip-toe around TempData, ChildActions, etc?
Well, the use of session state has mainly been an issue of scalability, but things are changing a bit with heavy use of Ajax in the browser. One big drawback of using Session State is that concurrent requests to session state within the same session must be done one at a time when done with full read-write access. You cannot access session state in parallel with full read-write privileges as data corruption can occur. Therefore if you have multiple, concurrent requests from the same session they will need to be performed one at a time rather than in parallel.
When does this become a big deal? Mainly when concurrent ajax requests from the browser are firing off in the background in the same user session. If session state is enabled with full read-write privileges, those scripts cannot be run in parallel as they could corrupt session state. They will be executed one-at-a-time and possibly deteriorate the responsiveness of the UI.
A way to get multiple, concurrent requests in the same session to perform in parallel would be to completely disable session state or make it read only when it is indeed read only.
Conclusion
A new feature in ASP.NET MVC 3 is the ability to specify session state behavior on controllers using the new [SessionState] Attribute. Although there are some challenges, they can easily be managed if you need to squeeze out every ounce of scalability and responsiveness from your application.
Hope this helps.