Let's say we are creating a simple form in our ASP.NET MVC 3 web application and there is a Body field on the form where we want to allow HTML Tags.
If we do not disable request validation in some manner for this Body field, we will get the dreaded error - A potentially dangerous Request.Form value was detected from the client (Body = "<br>").
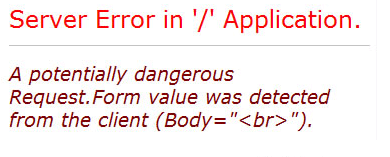
Request validation is a good thing since it keeps people from injecting script tags in our application for Cross-Site Scripting ( XSS ) attacks. However, in this case we want to disable request validation on the Body Field so we can put HTML in the body of our blog posts.
ValidateInput Attribute
In ASP.NET MVC 2 we used the ValidateInput Attribute on the action to disable request validation for the entire request
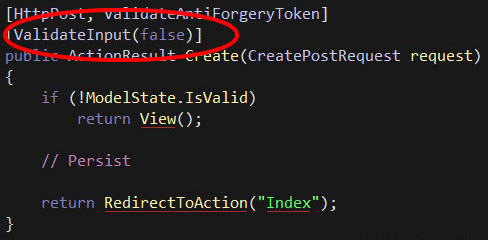
The downfall of this approach is that the ValidateInputAttribute disables request validation on all model properties, and we just want to disable request validation on a single property, called Body.
AllowHtmlAttribute in ASP.NET MVC 3
In ASP.NET MVC 3 we now have a property attribute that we can include on model properties to disable request validation on a property by property basis, called AllowHtmlAttribute. Instead of using the ValidateInputAttribute on the action, we turn off request validation just on Body by adding the [AllowHtml] Attribute to it:
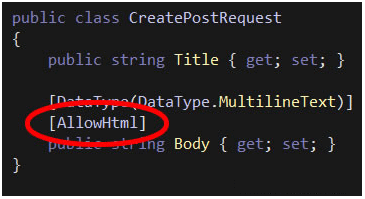
This allows HTML for the Body Property, but does not allow HTML for the Title Property, which is what we want.
[Note: Briefly in ASP.NET MVC 3, before it was released, there existed a SkipRequestValidationAttribute. It no longer exists and has been renamed to AllowHtmlAttribute.]